How to use switch case
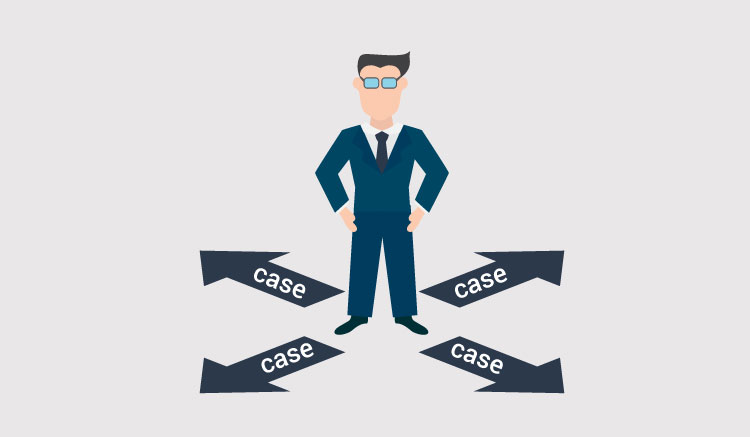
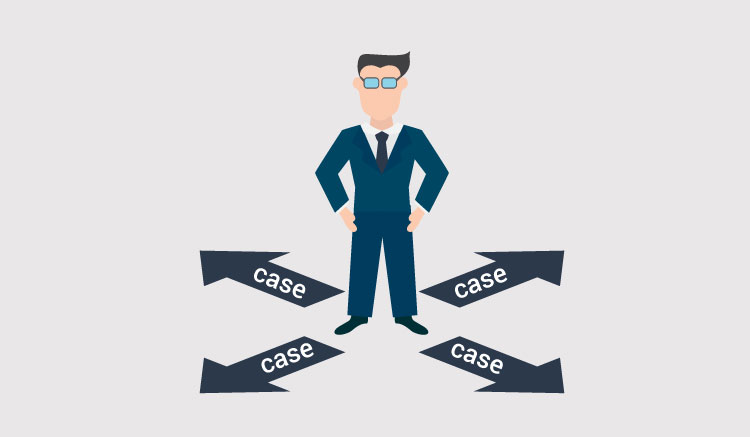
Let's make it simpler.
You use switch to say what variable you want to switch on and case to define what you want to do for each possible value. Be aware that if you don't put a break at the end of each case, the code will "fall through" to the next case statement. Sometimes that is desired behaviour, but should always be commented if it is. So:
switch (atoi (argv [1]))
{
case 1:
printf ("one\n");
break;
case 2:
printf ("two\n");
break;
default:
printf ("I can only count to 2\n");
break;
}
the switch statement will jump to the case which is matched or the default if no case is matched.
If you are going to use something like the example above, don't. Use a look up table instead:
const char *dow = {"Monday", "Tuesday"...};
printf ("It's %s\n", dow [i]);
}
Another Example:
include < stdio.h >
#include < conio.h >
#define N 4
int q[N];
int f=-1;
int r=-1;
void main()
{
int choice,x;
void qinsert(int);
int qdelete(void);
clrscr();
do
{
printf("\n1.Queue Insert");
printf("\n2.Queue Delete");
printf("\n3.Exit");
printf("\nEnter Your Choice:");
scanf("%d",&choice);
switch(choice)
{
case 1:
printf("\nEnter x:");
scanf("%d",&x);
qinsert(x);
break;
case 2:
printf("\n %d is Deleted",qdelete());
break;
case 3:
exit(0);
}
}while(choice!=3);
getch();
}
void qinsert(int x)
{
if(r > =N)
{
printf("\nOverflow");
getch();
exit(0);
}
r++;
q[r]=x;
if(f==-1)
f++;
}
int qdelete(void)
{
int x;
if(f==-1)
{
printf("\nUnderflow");
getch();
exit(0);
}
x=q[f];
if(f==r)
{
f=-1 ;
r=-1 ;
}
else
f++;
return(x);
}
This is a simple example of switch case.
Another Example:
include < stdio.h >
#include < conio.h >
#define N 4
int q[N];
int f=-1;
int r=-1;
void main()
{
int choice,x;
void qinsert(int);
int qdelete(void);
clrscr();
do
{
printf("\n1.Queue Insert");
printf("\n2.Queue Delete");
printf("\n3.Exit");
printf("\nEnter Your Choice:");
scanf("%d",&choice);
switch(choice)
{
case 1:
printf("\nEnter x:");
scanf("%d",&x);
qinsert(x);
break;
case 2:
printf("\n %d is Deleted",qdelete());
break;
case 3:
exit(0);
}
}while(choice!=3);
getch();
}
void qinsert(int x)
{
if(r > =N)
{
printf("\nOverflow");
getch();
exit(0);
}
r++;
q[r]=x;
if(f==-1)
f++;
}
int qdelete(void)
{
int x;
if(f==-1)
{
printf("\nUnderflow");
getch();
exit(0);
}
x=q[f];
if(f==r)
{
f=-1 ;
r=-1 ;
}
else
f++;
return(x);
}
This is a simple example of switch case.
No comments