Check Consecutive
Given an unsorted array of numbers, write a program that checks whether the array contains atleast 4 consecutive numbers or not, and returns true if the criterion meets. a) If array is {5, 2, 3, 1, 4}, then the function should return true because the array has consecutive numbers from 1 to 5.
b) If the array is {34, 23, 52, 12, 3 }, then the function should return false because the elements are not consecutive.
c) If the array is {7, 6, 5, 5, 3, 4}, then the function should return false because 5 and 5 are not consecutive.
Business Rules
Minimum length of the array should be 4. Else display "Invalid Length".
Maximum length of the array should be 50.Else display "Size Exceeded".
If array consists of negative element(s) in it, then display "Invalid List".
Input Format
Input consists of an unsorted integer array and it's size. Accept the size first followed by array elements. Refer sample input section for details.
Output Format
Display True if array consists of consecutive numbers, else display False. Refer sample output for details.
Function specification is the following for C#, Java and C++.
Name:- AreConsecutive
Return Type:- bool
Parameter(s):- int size,int arr[]
Function specification for C:
Name:- areConsecutive
Return Type:- bool [Include <stdbool.h> in your code for getting the reference of boolean]
Parameter(s):- int size,int arr[];
Sample Input 1:
5
4
3
2
1
5
Sample Output 1:
True
Sample Input 2:
65
Sample Output 2:
Size Exceeded
Sample Input 3:
3
Sample Output 3:
Invalid Length
Sample Input 4:
5
-6
3
2
1
0
Sample Output 4:
Invalid List
Sample Input 5:
5
69
87
12
36
78
Sample Output 5:
False
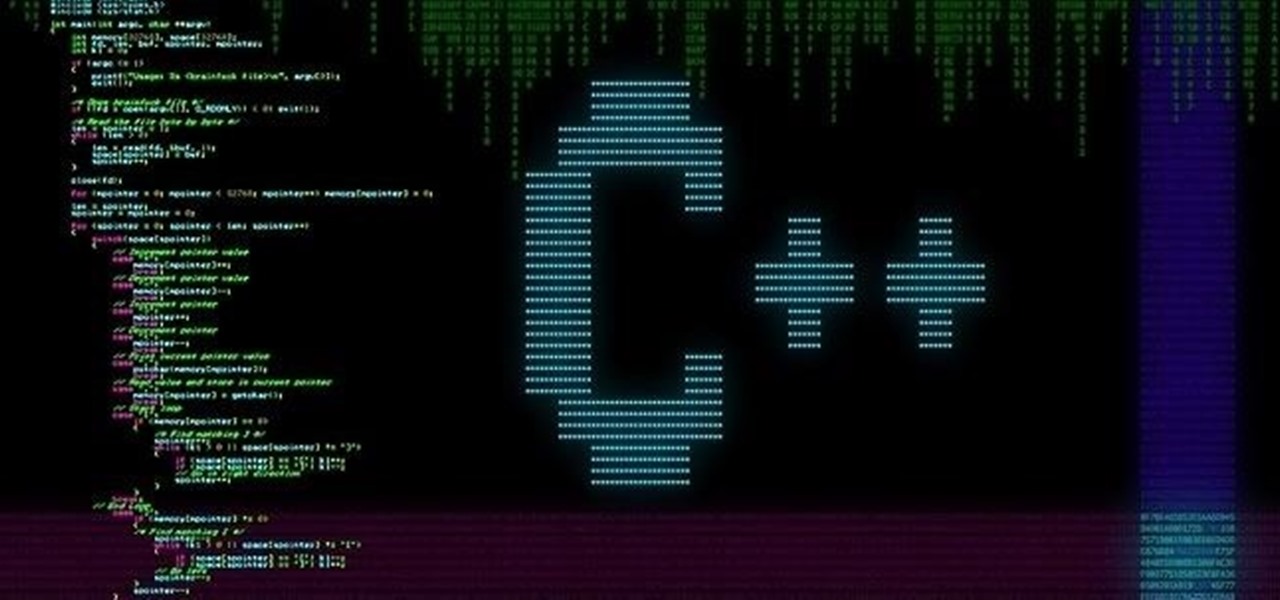
Code:
#include <bits/stdc++.h> using namespace std; /*Function which returns minimum value*/ int getMin(int arr[], int n) { int min = arr[0]; for (int i = 1; i < n; i++) if (arr[i] < min) min = arr[i]; return min; } /*Function which returns maximum value*/ int getMax(int arr[], int n) { int max = arr[0]; for (int i = 1; i < n; i++) if (arr[i] > max) max = arr[i]; return max; } /* function returns true if array elements are consecutive else returns false*/ bool ConsecutiveElements(int arr[], int n) { if (n < 1) return false; /* Get the minimum element in array */ int min = getMin(arr, n); /* Get the maximum element in array */ int max = getMax(arr, n); if (max - min + 1 == n) { for(int i = 0; i < n; i++) { int j; if (arr[i] < 0) j = -arr[i] - min; else j = arr[i] - min; /* if the value at index j is negative then there is repetition*/ if (arr[j] > 0) arr[j] = -arr[j]; else return false; } /* If we do not see a negative value then all elements are distinct */ return true; } // if (max – min + 1 != n) return false; } /* Driver program to test above functions */ int main() { int arr[]= {1, 4, 5, 3, 2, 6}; int n = sizeof(arr)/sizeof(arr[0]); if(ConsecutiveElements(arr, n) == true) cout<<" Array elements are consecutive "; else cout<<" Array elements are not consecutive "; return 0; }
No comments